Given the code fragment:
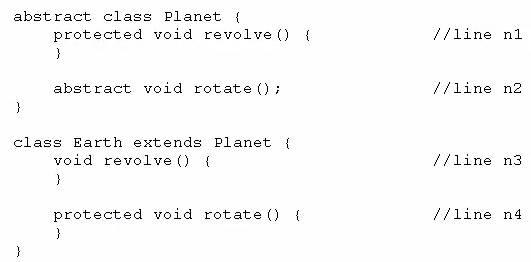
Which two modifications, made independently, enable the code to compile? (Choose two.)
Given the code fragment:
Which two modifications, made independently, enable the code to compile? (Choose two.)
To enable the code to compile, the methods in the subclass Earth that override methods from the superclass Planet must not have reduced visibility compared to those in the superclass. In Java, a method in a subclass cannot have less visibility than the method in the superclass that it overrides. Therefore, to ensure this requirement is met, making the method at line n3 public and the method at line n1 public will address this issue. By changing the visibility of the revolve method at line n3 to public, it ensures that it does not conflict with the protected visibility of the method being overridden. Similarly, making the method at line n1 public ensures uniform visibility necessary for overriding even though it's already called in an abstract class.
Given:
And given the code fragment:
What is the result?
The given code correctly uses the `super` keyword in the `Car` class's constructor to initialize the `Vehicle` class part of a `Car` object. The default values for `type` and `maxSpeed` fields in the `Vehicle` class are used when the no-argument constructor of the `Vehicle` class is called implicitly. Therefore, the result of the fragment is '4W 100 Auto' for the first instantiation and '4W 150 Manual' for the second instantiation, making the correct answer '4W 100 Auto 4W 150 Manual'.
Given:
What is the result?
The compilation fails at line n1 because the 'start()' method in class Caller is declared as private and thus cannot be accessed from outside the Caller class, including from the main method in the TestCall class. As a result, the compilation error will occur at the first encounter with this method call. The compilation will not reach line n2, hence the error associated with line n1 is the primary concern.
Given these two classes:
Any amount of electricity used by a customer (represented by an instance of the Customer class) must contribute to the customer's bill (represented by the member variable bill) through the useElectricity method.
An instance of the Customer class should never be able to tamper with or decrease the value of the member variable bill.
How should you write methods in the ElectricAccount class at line n1 so that the member variable bill is always equal to the value of the member variable kwh multiplied by the member variable rate?
A.
B.
C.
D.
The correct answer should be option B. This method ensures that only positive values of kWh are added to the customer's ElectricAccount and prevents the possibility of decrementing the value, thereby maintaining the integrity of the bill. The method `addKWh` checks if the `kWh` parameter is greater than 0 before adding it to the account's `kWh` and updating the bill accordingly. This approach ensures that negative values do not tamper with the `bill` variable, adhering to the requirement that an instance of the Customer class should never be able to tamper with or decrease the value of the member variable bill. Therefore, option B is the best choice as it provides the necessary validation and protection of the `bill` variable.
Given the code fragment:
What is the result?
The code first converts the StringBuilder object 'sb' to a String using the toString() method and then compares it with the String 's' using the equals() method. Since both 'sb.toString()' and 's' have the value 'Java', the equals() method returns true, and 'Match 1' is printed.