Given a java source file:
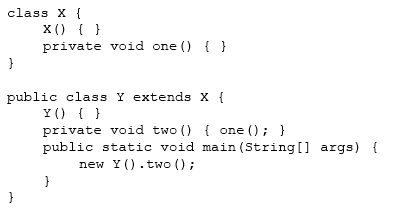
What changes will make this code compile? (Select Two)
Given a java source file:
What changes will make this code compile? (Select Two)
To ensure the code compiles correctly, two changes need to be made. First, the method 'one()' in class X should be accessible from class Y, which can be achieved by changing the 'private' modifier on the 'one()' method to 'protected'. This allows class Y, being a subclass of class X, to access the 'one()' method. Second, the constructor of class X should be accessible when an object of class Y is created. Adding the 'protected' modifier to the constructor X() will allow this, as it will be accessible to the subclass Y. Therefore, the 'protected' modifier should be added to the 'x()' constructor and the 'one()' method.
Given:
What three modifications, made independently, made to class greet, enable the code to compile and run?
Line 6 in the given code needs to access the full path of the Keystroke class in the handy.dandy package. To enable the code to compile and run, we can either replace line 6 with the full path to the Keystroke class and its creation, which is 'handy.dandy.KeyStroke stroke = new handy.dandy.KeyStroke();', or we can import the package containing the Keystroke class. The modifications that work are: (1) replacing line 6 with 'handy.dandy.KeyStroke stroke = new handy.dandy.KeyStroke();', (2) adding the import statement 'import handy.*;' before line 1, which will import all classes in the handy package including any sub-packages, and (3) adding the import statement 'import handy.dandy.*;' after line 1, which will specifically import all classes in the handy.dandy package.
Given the code fragment:
What is the result?
When execution reaches the switch statement, it evaluates the expression and starts executing the matching case. In this fragment, the value of the 'color' variable is 'Red,' so the case 'Red': statement is executed. However, since there is no 'break' statement following the 'System.out.println("Found Red");', the execution continues to the next case 'Blue': and then 'System.out.println("Found Blue");' is executed as well. Thus, the output is 'Found Red' followed by 'Found Blue'.
Given:
class X {}
class Y { Y ( ) { } }
class Z { Z (int i ) { } }
Which class has a default constructor?
A default constructor is a constructor that is automatically created by the compiler if no other constructors are defined. It typically has no parameters. In this case, class X has no constructors defined explicitly, so it has a default constructor. Class Y has a constructor defined, but since it takes no parameters, it can be considered a default constructor as well. Class Z has a parameterized constructor, which means it does not have a default constructor. Therefore, classes X and Y have default constructors.
Given:
What is the result?
The code provided has a compilation error. Specifically, there is no closing bracket for the class 'DoCompare4'. Additionally, each 'while' statement should be followed by an expression in parentheses. The second 'while' should not have a semicolon after the parentheses if it is intended to be a part of the do-while loop. Therefore, the code will fail to compile.