Given:
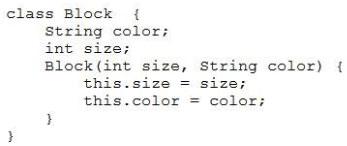
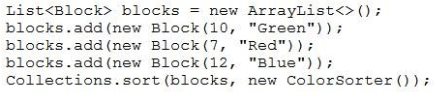
Which definition of the ColorSorter class sorts the blocks list?
A.
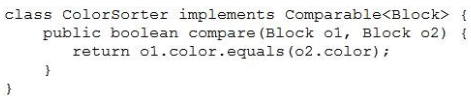
B.
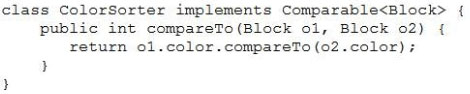
C.

D.
Given:
Which definition of the ColorSorter class sorts the blocks list?
A.
B.
C.
D.
The correct definition for the ColorSorter class that sorts the blocks list is option C. This option implements the Comparator interface, which requires implementing the compare method that takes two Block objects as parameters and returns an integer. The implementation compares the color attributes of the Block objects using the compareTo method of the String class, which is the appropriate way to compare two strings lexicographically. Therefore, option C is the correct answer.
Answer is C, import java.util.*; class Block { String color; int size; public Block(int size, String color) { this.color = color; this.size = size; } @Override public String toString() { return "Block{" + "color='" + color + '\'' + ", size=" + size + '}'; } } class ColorSorter<B> implements Comparator<Block> { public int compare(Block o1, Block o2) { return o1.color.compareTo(o2.color); } } public class Test { public static void main (String[] args) throws InterruptedException { List<Block> blocks = new ArrayList<>(); blocks.add(new Block(10, "Green")); blocks.add(new Block(7, "Red")); blocks.add(new Block(12, "Blue")); Collections.sort(blocks, new ColorSorter()); System.out.println(blocks); } }
c is correct. Tested
C is true. With Comparator you must override public int compare(Block b1, Block b2) method.