Given the definitions of the Bird class and the Peacock class:
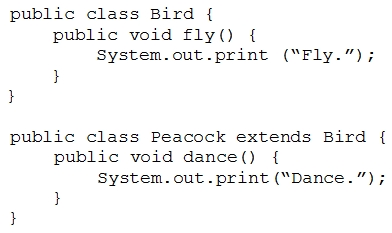
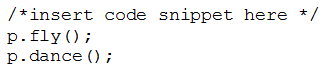
Which code snippet can be inserted to print Fly.Dance. ?
Given the definitions of the Bird class and the Peacock class:
Which code snippet can be inserted to print Fly.Dance. ?
To achieve the desired output where both methods from the Bird and Peacock classes are invoked correctly, the object must first be created as a Peacock to ensure that its methods are available. Then, it is cast back to a Peacock to allow direct method calls specific to the Peacock class. Hence, the correct code snippet is: Bird b = new Peacock(); Peacock p = (Peacock) b; This allows p to access both the fly() method from Bird and the dance() method from Peacock, resulting in the output 'Fly.Dance.'.
Correct answer is D. For option B there is ClassCastException as class Bird cannot be casted to class Peacock
Tested. Answer is D. To test: class Bird { public void fly() { System.out.print("Fly."); } } class Peacock extends Bird { public void dance() { System.out.print("Dance."); } } public class Test { public static void main(String[] args) { Bird b = new Peacock (); Peacock p = (Peacock) b; p.fly(); p.dance(); } }
Answer is D. class Bird{ public void fly() { System.out.println("fly"); } } public class Peacock extends Bird { public void dance() { System.out.println("dance"); } public static void main(String[] args) { Bird b= new Peacock(); Peacock p= (Peacock) b; // Bird b= new Bird(); // Peacock p= (Peacock) b; // ClassCastException p.fly(); p.dance(); } }
Tested. Answer is - D. Bird b = new Peacock (); Peacock p = (Peacock) b;
I tested it. For B option is taken cast exception. D option worked correctly..
It's not B because with Bird B = new Bird() and peacock p = (peacock) B; it's as if this becomes peacock p = new bird(); which is wrong
only D
The correct answer is the letter B, you need to create an object B and then do a casting for it, making the same be able to access the method of the child class.
You're are wrong. Correct answer is D. Option B is not how downcasting works. Option D is the textbook definition of downcating. With option our object type is peacock and class type of reference variable is also Peacock and peacock is a child class. Child class peacock can access it's method as well as Parent class Bird method.
*With option D