Given:
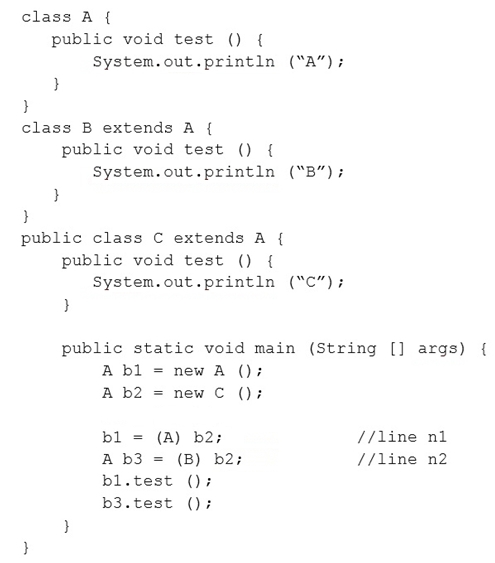
What is the result?
Given:
What is the result?
In the given code, class B and class C are both subclasses of class A, but there is no inheritance relationship between class B and class C. Therefore, you cannot cast an instance of class C to class B. When line n2 is executed, a ClassCastException will be thrown because b2, which is an instance of class C, cannot be cast to class B. Therefore, the correct result is that a ClassCastException is thrown at line n2.
The right answer is the letter E, class C cannot be B because B extends A and C also extends C, there is no inheritance between C and B because neither of the 2 classes extends the other, generating the exception Class Cast Exception on the line 2 where trying to perform the polymorphism of C -> B. I also tested the code, if you want to test it too it is below: class A { public void test () { System.out.println ("A"); } } class B extends A { public void test () { System.out.println ("B"); } } public class C extends A { public void test () { System.out.println ("C"); } public static void main(String[] args) { A b1 = new A (); A b2 = new C (); b1 = (A) b2; //line n1 A b3 = (B) b2; //line n2 b1.test (); b3.test (); } }
Answer is E as C cannot be cast to B as it does not inherit from that class
E : B is subclass of A, C is subclass of A, we can cast from one to another via implicite and explicite casting C is not a subclass of B, so we can't cast from C to B and vice versal
Answer is C C tested
Answer is E
The answer is E
Tested as below, with iSnover's source code, answer is E.
Answer is E. It shows the error as " Exception in thread "main" java.lang.ClassCastException: class p1.Acc cannot be cast to class p1.B (p1.Acc and p1.B are in module KK.java of loader 'app') at KK.java/p1.Acc.main(Acc.java:20) "