Given the code fragment:
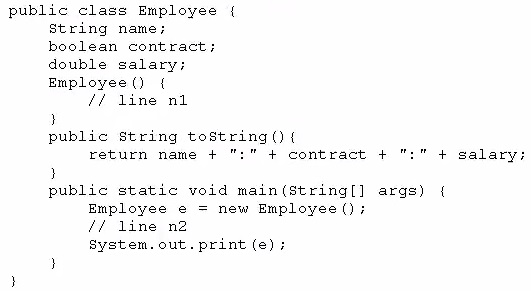
Which two modifications, when made independently, enable the code to print joe:true: 100.0? (Choose two.)
Given the code fragment:
Which two modifications, when made independently, enable the code to print joe:true: 100.0? (Choose two.)
The code aims to print 'joe:true: 100.0' when executed. For this output, the `name` field must be 'Joe', the `contract` field must be `true`, and the `salary` field must be 100. Option A correctly sets these values after creating the `Employee` object by directly assigning values to its fields, which is valid. Option D also correctly sets these values but within the constructor, again ensuring that the fields have the required values when the `toString` method is called. Options B and C are incorrect because `this` cannot be used in a static context, and Option E is syntactically incorrect because it attempts to use a constructor that doesn't exist in the given class definition.
AC or AD - both correct
Tested. Answer is A and C. Comments: * Option C works even though it has been deprecated since version 9 (1z0-808 is about java 8). ** Option B would only be correct if it is inside the constructor, on line n1. As option B is pointing to line n2, it will throws an error.
Here is the code for testing: public class Employee { String name; Boolean contract; double salary; Employee(){ // Line n1 - Option C /* this.name = new String("Joe"); this.contract = new Boolean(true); this.salary = new Double(100); */ } public String toString() { return name + " : " + contract + " : " + salary; } public static void main(String [] args) { Employee e = new Employee(); // Line n2 - Option A /* e.name = "Joe"; e.contract = true; e.salary = 100; */ // Line n2 - Option B /* this.name = "Joe"; this.contract = true; this.salary = 100; */ System.out.print(e); } }
Should be A, C. We cannot do B as we cannot use "this" in the static method
AC the last line in main method should be : System.out.print(e.toString();
Answer - A and C
A is correct, C is incorrect, boolean is not same as Boolean, so this.contract = new Boolean("True"); is a compilation error for. D can be the another correct option if we are writing true instead of TRUE. Other than this none of these are correct.
Why is D not correct?
Because its true and not TRUE.
Respuesta correcta es A, recordar que esta en un cotexto estatico por lo que no puede acceder con this ni menos con con la nombre de la variable
A and C
a and c are correct
The correct options are A and C, as all others contain syntax errors. It cannot be the letter B because "this" cannot be instantiated in static methods.
If C, unnecesary boxing to Boolean and Double. If we use "new" we will create new objects.
Wrong, you can't put "this" in static methods. The correct one is A and C.
wrong, it is A, C and D