You need to write some Terraform code that adds 42 firewall rules to a security group as shown in the example.
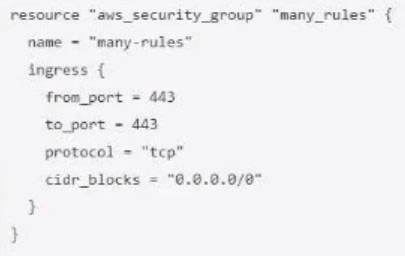
What can you use to avoid writing 42 different nested ingress config blocks by hand?
You need to write some Terraform code that adds 42 firewall rules to a security group as shown in the example.
What can you use to avoid writing 42 different nested ingress config blocks by hand?
A dynamic block in Terraform is used to generate multiple nested blocks based on the information provided in a loop, which is ideal for creating several similar sub-blocks without repeating code. This is particularly useful for avoiding the manual creation of multiple nested blocks, such as writing 42 different ingress blocks for a security group. By iterating over a collection of input values, a dynamic block can programmatically generate each nested ingress block, making the code more maintainable and concise.
Answer is D. A dynamic block acts much like a for expression, but produces nested blocks instead of a complex typed value. It iterates over a given complex value, and generates a nested block for each element of that complex value. Reference: https://www.terraform.io/language/expressions/dynamic-blocks
resource "aws_security_group" "many_rules" { name = "many_rules" dynamic "ingress" { for_each = var.ingress_rules content { from_port = ingress.value.from_port to_port = ingress.value.to_port protocol = ingress.value.protocol cidr_blocks = ingress.value.cidr_blocks } } } variable "ingress_rules" { description = "A list of ingress rules" type = list(object({ from_port = number to_port = number protocol = string cidr_blocks = list(string) })) default = [ { from_port = 80 to_port = 80 protocol = "tcp" cidr_blocks = ["0.0.0.0/0"] }, # Add 41 more rules here... ] }
https://www.terraform.io/language/expressions/dynamic-blocks
Some additional information comparing each of the options: https://awstip.com/terraform-for-vs-for-each-7ff8506a1f94 Answer is definitely D as the question specifically mentions 'nested config(uration) blocks'
https://developer.hashicorp.com/terraform/tutorials/configuration-language/count
yep, D is correct.