Take a look at the snippet, and choose the true statements: (Choose two.)
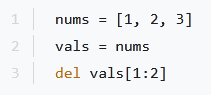
Take a look at the snippet, and choose the true statements: (Choose two.)
Initially, both nums and vals point to the same list [1, 2, 3]. When using 'del vals[1:2]', we remove the second element from vals, but since nums and vals refer to the same list, it also affects nums. Now, both nums and vals will be [1, 3]. Hence, nums is longer than vals is incorrect, as they are of the same length. nums and vals are of the same length is incorrect because they both have the same elements after deletion. vals is longer than nums is incorrect because both lists are the same length. The correct statements are nums is longer than vals (A) and nums and vals refer to the same list (D).
What is the output of the following snippet?
The given code creates a dictionary with two keys: '1' and '2'. The value associated with '1' is a tuple (1, 2) and the value associated with '2' is a tuple (2, 1). The for loop iterates over the keys of the dictionary, and for each key, it prints the second element of the tuple associated with that key. Therefore, it prints the second element of (1, 2) which is 2, and then the second element of (2, 1) which is 1. The output is '21'.
What is the expected output of the following code?
print(list('hello'))
The expected output of the code print(list('hello')) is ['h', 'e', 'l', 'l', 'o']. When the list() function is used on a string, it converts the string into a list of its individual characters, thus producing a list where each element is a single character from the string.
What will be the output of the following code snippet?
The code snippet utilizes slicing on a list with the notation a[::2]. This syntax means starting from the beginning of the list, the step is 2, so it will take every second element of the list. Therefore, the elements that would be picked are [1, 3, 5, 7, 9], which corresponds to option A.
What will be the output of the following code snippet?
In the given code, a dictionary (d) is created and two key-value pairs are added: one with the integer key 1 and another with the string key '1'. Python distinguishes between the integer and string keys, so they are treated as separate entries. The subsequent operation increments the value associated with the integer key 1 by 1. The dictionary thus becomes {1: 2, '1': 2}. The sum is then initialized to 0, and a for loop iterates over the keys in the dictionary, adding their values to the sum. The final sum is the sum of 2 and 2, which equals 4. Therefore, the correct output is 4.